Apple’s Swift and What it Means for Developers and Users
by Brandon Chester on June 11, 2014 8:00 AM EST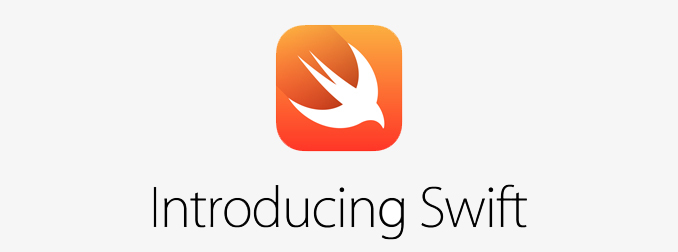
On Monday Apple revealed iOS 8 and OS X 10.10 Yosemite. Both of these were expected by everyone who has followed Apple’s pattern of software reveals at WWDC for the past few years. But Apple also revealed new power tools for developers that nobody had expected or even imagined would arrive. One of the most significant of these is Apple’s new Metal API to greatly improve graphic performance by removing the limitations and performance impacts created by using OpenGL. Another, and arguably the most significant of them all, is Swift. Swift is Apple’s new programming language. After four years in development and without a single leak, Apple has created and now revealed what will become the foundation of a new generation of applications for both OS X and iOS.
Safety, modern syntax, and powerful features are three design principles that guided the development of Swift and it really shows. There are huge benefits for developers which translate to benefits for users. Naturally because of that, the first thing to discuss is why Swift is great for programmers before discussing why it will be great for users.
Modern Syntax for Modern Code
Being a brand new language, Swift strives to have a modern syntax that enhances code legibility, and works against bad programming habits. One of the most significant of these syntax changes is the elimination of the use of semicolons at the end of statements. For those who are unfamiliar with programming syntax, a statement essentially states an action to be performed. For example, the statement “X = 5;” would mean to set the variable X to be the number five. In many languages such as C, Java, Flash ActionScript, and of course Apple’s own Objective-C every statement must be ended with a semicolon. In Swift, Apple goes for an approach similar to languages like Python where it is not necessary to end statements with a semicolon. While this may be a difficult change to get used to for programmers used to programming in Objective-C, it will greatly reduce the frustration involved with errors due to the programmer simply forgetting to insert the semicolon at the end of a statement.
Another massive improvement comes in the form of new variable declarations and definitions. Traditionally in programming languages a variable is defined by calling it a variable, setting a name, setting a type, and setting a value. For example, the line “var year: = int “2014”;” indicates that a variable named year is being created and that it is an integer with a value of 2014. In Swift Apple has included support for type inference. Essentially, it is no longer necessary for a programmer to indicate what type of variable they are declaring. Based on what the variable is being declared as, Swift is able to infer what type of variable it should be. This means that the previously mentioned line can be shortened to simply read “var year: = “2014”.” This applies to any variable types and also extends to data structures such as arrays where an array of names can simply be defined as var names = ["Elaine", "Daniel", "Jash"] and Swift will know that it is an array of strings.
Now of course, the word variable implies that the programming is making a value they intend to change. But what if the programmer is declaring, for example, an integer that should always remain constant? In Swift the programmer is able to define an immutable constant by using the definition “let” rather than “var”. This is a big part of Swift’s new syntax. Immutability is preferred for values that are not going to be changed. From a visual standpoint, defining a constant makes it very clear to anyone reading over code which values are being altered and which are not. From a performance standpoint, declaring values as constants allows the compiler to better optimise code as it knows which values will be changed and which will remain the same. There are also safety benefits to this which will be touched on more later.
There is one final thing to touch on relating to the new code syntax. With Swift, a programmer can assign essentially any Unicode character to be the name of a variable. This allows the use of symbols like the Greek letter theta to represent an angle which further improves readability and effectively communicates what a variable is intended to be. In addition, it also supports emoji and so programmers can assign an emoticon of a banana to represent the word banana should they see fit.
The Programming Power Tools
Swift wouldn’t be a brand new language without some brand new tools and abilities for programmers to take advantage of. Any programmer who uses Apple’s Foundation Framework will be pleased to know that in Swift all typical variables are able to make sure of Foundation methods, and all methods that return a variable return a standard Swift variable rather than an NS variable.
Swift also includes many new ways to modify variables. With Swift strings, a programmer is able to append characters to the end simply by adding them much like accumulating one integer into another. For example, if the programmer takes the string “var name: = “Elain”” and the character “var end: = “e”” they may then write the statement “name += end” and the string will become “Elaine”. This is a stark contrast to Objective-C which would require the use of the NSMutableString type and much more code. This method also works for other data types. Strings may be added together, and arrays can also have values or even sets of values added. If any of the objects are defined using “let” rather than “var” the compiler will give an error about attempting to alter immutable objects.
One of the most powerful features of Swift is something Apple is calling Generics. Generics allow the programmer to create a function that performs a generic task that can work for multiple variable types. For example, a programmer may create a function that adds two variables together, and they may be integers, doubles, strings, etc. The programmer can create a function with a placeholder type name enclosed in angle brackets after the function definition. All variables passed to the function will be created under the placeholder type and the addition operation will be performed on them. This allows the programmer to have a generic piece of code that can perform the same operation on whatever variable types are passed to it, rather than having a function that is limited to accepting a single type of variable.
Safety
One of the big points emphasized with Swift is that it’s safe. Apple’s SSL bug that was revealed earlier this year is a prime example of how such a small code issue can be catastrophic and how it can be missed for quite some time. The explanation of Apple’s SSL bug is rooted in programming syntax. Traditionally when a programmer writes an “if statement” they are evaluating a statement to see if it is true or not. If it is true, the code contained within the if statement is run. Typically an if statement is opened and closed with brace brackets to designate what code is within it. However, in some programming languages if the if statement is resolved in one line it is not necessary to put brace brackets. This is where the security issue arises. When written with brace brackets, the part in the above code where the error occurs should read like this.
if ((err = SSLHashSHA1.update(&hashCtx, &signedParams)) != 0) {
goto fail;
}
goto fail;
As you can see, no matter what occurs with the if statement, the second goto fail line is always reached and the SSL verification is terminated. While this bug was likely created by some sort of accident relating to copying and pasting code, it would have been much easier spotted during review had the brace brackets been there to show how the code actually should appear. Clearly Apple is aware of this sort of issue and with Swift they have addressed it. In Swift the programmer must always include brace brackets to open and close an if statement which prevents a whole slew of bugs that can occur due to what is honestly just sloppy programming practices.
Another safety aspect relates to something touched on earlier which is immutability. In programming there is a concept known as thread safety which requires shared data structures to be altered in such a way that execution by multiple threads at the same time will not cause errors. With Swift pushing for all objects that will not be altered to be declared as immutable constants they ensure thread safety for those specific pieces of data as they are not being altered and can be executed in code on multiple threads safely. Swift also ensures that you know what type of data is held in variables and data structures. For example, in the aforementioned array of strings var names = ["Elaine", "Daniel", "Jash"] Swift would throw an error should the programmer attempt to add anything other than a string to the array.
One of the final safety related features relates to switch statements. Switch statements can function in a similar way to if statements in that they test a variety of possible scenarios. If implemented poorly this can open the door for code safety issues if a programmer creates a switch statement that does not satisfy any of the given possibilities. For this reason, Swift requires that all switch statements include a default statement which will be run if all other cases fail.
What This Means for Users
Anyone reading this who is not a programmer may be wondering how this impacts them as a user. Well in fact, all of these changes that Apple has made to make things easier for developers also make things easier for users. Apple’s improvements to syntax will eliminate a great deal of time that developers spend resolving small bugs that are hard to spot and relate to a silly issue like a missing bracket or the semicolons that cause annoyance to no end. This means that a developer can find and resolve issues faster which in turn means faster releases and updates for the user. It also means the developer can spend less time debugging and more time doing what they want to do which is add and improve the features in their applications.
Apple’s new tools and methods for writing code allow developers to perform actions more easily and with shorter, more concise code. For the end user this could potentially mean new features that had been more difficult to implement in the past, and it most certainly means that apps will be faster than ever before. In fact according to Apple, Swift can be nearly twice as fast as Objective-C in complex sorting tasks and in performing encryption.
Apple’s safety improvements also serve to benefit the user by preventing issues with incorrect data input that the programmer may not have foreseen. By trying to protect the language from common programming errors relating to variable mutability, thread safety, and input, the user is much less likely to encounter a scenario where a crash occurs in an application.
We’re still learning about Swift. According to Apple 370,000 people downloaded the handbook on the iBooks Store only a day after it was unveiled at the WWDC Keynote. As people work through the handbook and begin to create new applications and augment their existing ones using this powerful new language both the users and Apple themselves will finally see the fruits of their four year labor. For anyone interested in learning more about Swift and what it offers you can download the official Swift handbook on the iTunes store.
79 Comments
View All Comments
maxiniu - Wednesday, June 11, 2014 - link
Except for the Unicode variable names (which is nice, but not extremely important), all the features heralded in this article exist in C# for years. Needless to say, when writing Windows Phone apps, you can use C#...lmcd - Wednesday, June 11, 2014 - link
Well, and the syntax uses semicolons (which is a good thing, as it also improves readability)DERSS - Wednesday, June 11, 2014 - link
Semicolons, even though are not a requirement, are allowed on Swift, so if anyone wants to use them, it is possible.rkcth - Friday, June 13, 2014 - link
Thank goodness. I hate languages where you can't use semicolons, it leads to strange errors sometimes and reduces readability in my opinion though even allowing no semi-colons is a bad thing in my opinion. For example a really long SQL query where you might be concatenating multiple strings can get messy if you don't break it into multiple lines, but then because its a new line the language may misinterpret the code. I'm not a huge fan of non-semi colon languages for this reason. C# is the best designed language I have used and I've used a LOT of languages (last I had counted I was close to 25). With that said this sounds like a nice improvement over objective c. My biggest beef with programming on Macs though is the way the HOME, END, Page Up, Page Down, shift and control work. I use those keys in various combinations to copy and paste lines of code very rapidly without thinking about it. When I get on a mac it slows me way down and there appears to be no way to do many very powerful keyboard based selection commands that I take for granted on Windows. It slowed me down so much that I actually gave up writing an app I was making a while back and sold the mac mini I was coding it on. When you get used to programming at a certain speed it makes it tough to take that change. I wish you could develop iphone apps on windows and still use apple's tools, but I understand why they don't support that.ex2bot - Wednesday, June 11, 2014 - link
I hope you're not proposing that Apple users / developers solve the problem of imperfect development tools by switching to Windows. Cause that would be control freakish (freakery?), right. :)name99 - Wednesday, June 11, 2014 - link
True except for the single MOST important point --- Swift interoperates with the Objective C runtime and Objective C semantics, and C# does not...Look, Swift is not MEANT to change the world of programming as we know it. It is APPLE's solution to APPLE DEVELOPERs' problems. Complaining that it doesn't solve your problems, or that something else would be nicer (even though it doesn't actually solve Apple's problems), or that is has no new concepts ALL kinda miss the point.
vFunct - Friday, June 13, 2014 - link
Too bad C# is a slow-as-hell byte code interpreted language like Java, instead of an actual compiled language like C/C++/Objective-C.rkcth - Friday, June 13, 2014 - link
C# is not slow at all, its insanely fast, and due to the abundant and high quality libraries included, you can actually develop much more complex solutions easily, and refactor them to much bigger picture optimizations where needed.winterspan - Friday, June 13, 2014 - link
This is completely incorrect. C# uses byte code (MSIL) but it is not interpreted; it has a very performant JIT compiler.The runtime safety (like bounds checking arrays and garbage collection) is a very small CPU overhead, although a bit more in memory.
Given proper technique, C# applications can run 95+% the speed of C++. Similarly, you can also use pointers and disable bounds checking for the routines that need to be even more optimized.
Also, Microsoft recently released a new compiler that allows the code to be compiled to native code instead of MSIL.
Finally, one of the most popular gaming frameworks on iOS is Unity, and one of the most popular cross platform mobile development frameworks is Xamarin, and both solutions create incredibly fast iOS games and applications.
V900 - Monday, June 16, 2014 - link
Don't forget, that when developing WindowsPhone apps in C# you don't need to worry about pesky issues like monetization or marketshare either!Unlike Apple, that like a true control freak, insists on dragging their developers through the problems of dealing with a huge market of potentially paying customers, Microsoft has made a truly developer friendly platform, with an potential market so small that monetization is Impossible.
That leaves developers free to spend all their time doing what they do best: Developing!